Angular for React Developers: Bridging the Framework Gap
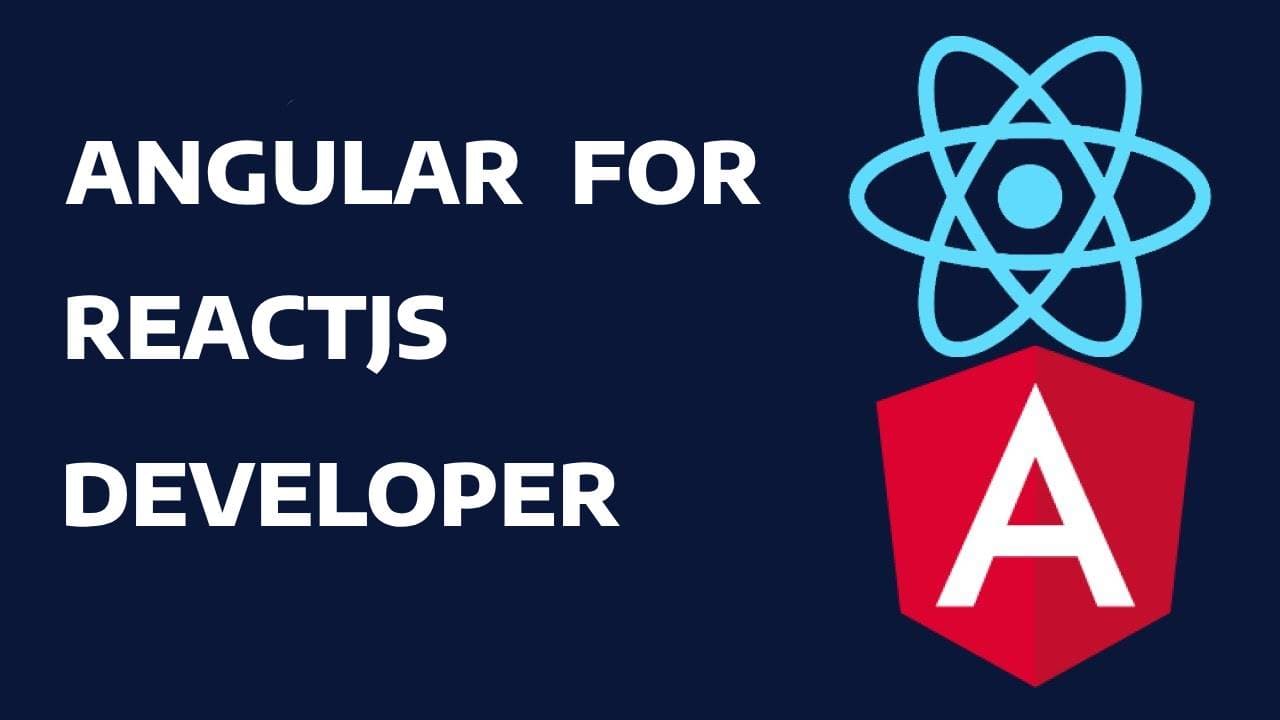
Table Of Content
- Understanding the Transition from React to Angular
- Key Differences to Understand
- Component Structure
- State Management Comparison
- React (using Hooks)
- Angular (Signals)
- Angular 18's Game-Changing Features for React Developers
- 1. Signals: React's useState Reimagined
- 2. Standalone Components
- 3. Enhanced Reactive Forms
- Migration Strategies
- Step-by-Step Transition
- Performance Considerations
- Common Challenges and Solutions
- Handling Async Operations
- Dependency Injection
- Conclusion
- Recommended Learning Path
Understanding the Transition from React to Angular
As a React developer, diving into Angular might seem like entering a new world. This guide will help you leverage your existing JavaScript knowledge to quickly adapt to Angular's ecosystem and powerful features.
Key Differences to Understand
Component Structure
In React:
function UserProfile({ name, email }) {
return (
<div>
<h1>{name}</h1>
<p>{email}</p>
</div>
);
}
In Angular :
@Component({
selector: 'app-user-profile',
template: `
<div>
<h1>{{ name }}</h1>
<p>{{ email }}</p>
</div>
`
})
export class UserProfileComponent {
@Input() name: string;
@Input() email: string;
}
State Management Comparison
React (using Hooks)
const [users, setUsers] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetchUsers().then(data => {
setUsers(data);
setLoading(false);
});
}, []);
Angular (Signals)
@Component({
selector: 'app-user-list',
template: `
@if (loading()) {
<p>Loading...</p>
}
@for (user of users(); track user.id) {
<div>{{ user.name }}</div>
}
`
})
export class UserListComponent {
users = signal<User[]>([]);
loading = signal(true);
constructor(private userService: UserService) {
this.userService.getUsers().pipe(
tap(data => {
this.users.set(data);
this.loading.set(false);
})
).subscribe();
}
}
Angular 18's Game-Changing Features for React Developers
1. Signals: React's useState Reimagined
Angular introduces Signals, a reactive primitive similar to React's useState, but with more granular reactivity:
- Fine-grained reactivity
- Automatic dependency tracking
- Performance optimizations
- Easier state management
2. Standalone Components
Angular embraces a more React-like component philosophy:
@Component({
standalone: true,
selector: 'app-dashboard',
imports: [CommonModule, ChartsModule],
template: `
<div>Dashboard Content</div>
`
})
export class DashboardComponent {}
3. Enhanced Reactive Forms
Simplified form handling that feels more intuitive:
@Component({
template: `
<form [formGroup]="loginForm" (ngSubmit)="onSubmit()">
<input formControlName="username" />
<input formControlName="password" type="password" />
<button type="submit">Login</button>
</form>
`
})
export class LoginComponent {
loginForm = new FormGroup({
username: new FormControl('', Validators.required),
password: new FormControl('', Validators.required)
});
onSubmit() {
if (this.loginForm.valid) {
// Handle login
}
}
}
Migration Strategies
Step-by-Step Transition
- Learn TypeScript fundamentals
- Understand Angular's dependency injection
- Practice with standalone components
- Leverage RxJS for state management
- Explore Angular CLI for project setup
Performance Considerations
- Angular offers built-in performance optimizations
- Ahead-of-Time (AOT) compilation
- Tree-shaking capabilities
- Improved change detection
Common Challenges and Solutions
Handling Async Operations
React developers will find RxJS similar to Promise-based workflows:
// RxJS Observable
this.users$ = this.userService.getUsers().pipe(
map(users => users.filter(user => user.active)),
catchError(error => of([]))
);
Dependency Injection
A powerful Angular feature absent in React:
@Injectable({
providedIn: 'root'
})
export class UserService {
constructor(private http: HttpClient) {}
getUsers(): Observable<User[]> {
return this.http.get<User[]>('/api/users');
}
}
Conclusion
Angular offers React developers a robust, opinionated framework with powerful built-in tools. While the learning curve might seem steep, the structured approach and comprehensive ecosystem can significantly enhance your web development capabilities.
Recommended Learning Path
- Official Angular documentation
- Online courses specializing in Angular
- Build small projects to gain practical experience
- Join Angular community forums
Embrace the learning journey, and you'll find Angular to be a powerful addition to your web development toolkit!