Typescript
Google AdSense in Next.js Applications 2024
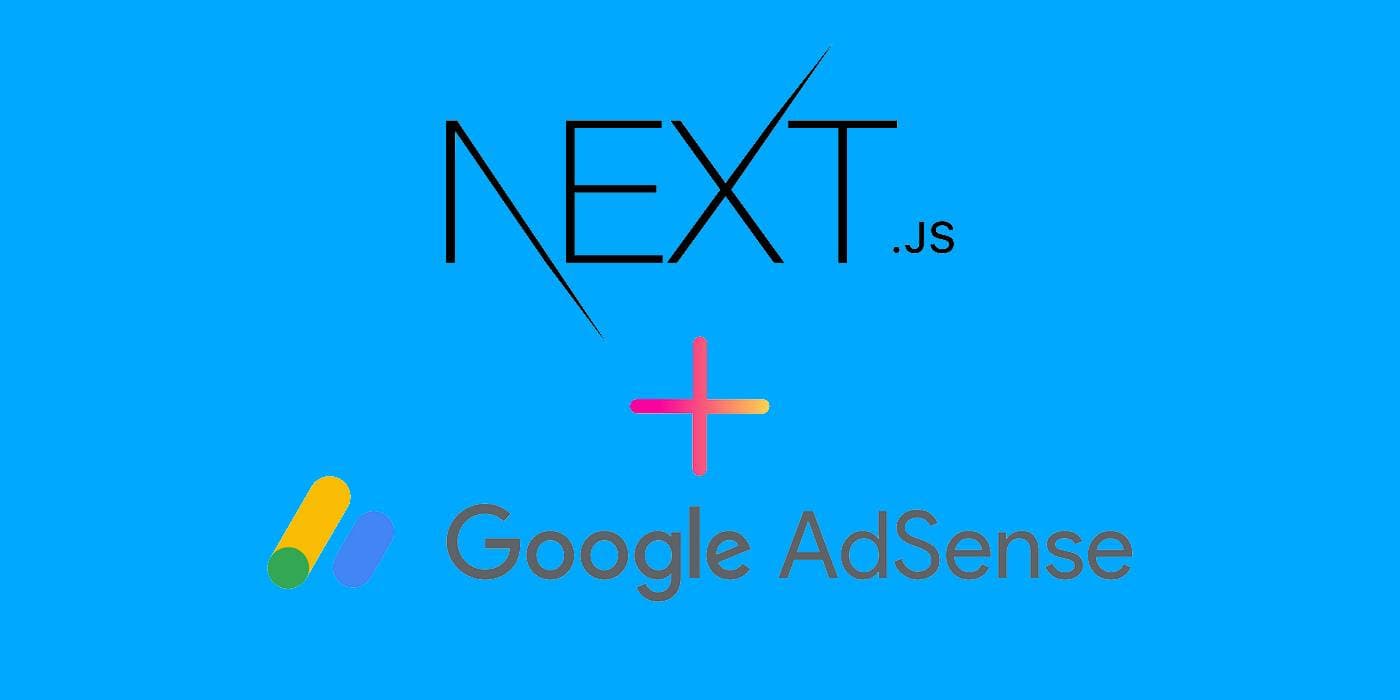
Implementing Google AdSense in Next.js Applications
Google AdSense is a powerful tool for monetizing your Next.js website. This comprehensive guide will walk you through the process of integrating AdSense into your Next.js application.
Prerequisites
Before starting, ensure you have:
- An approved Google AdSense account
- A Next.js project (version 13 or higher)
- Basic understanding of Next.js and React
Step-by-Step Integration
1. Adding the AdSense Script
First, add the AdSense script to your application. In Next.js, modify your pages/_document.tsx
file:
import { Html, Head, Main, NextScript } from 'next/document'
export default function Document() {
return (
<Html>
<Head>
<script
async
src="https://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js?client=ca-pub-XXXXXXXXXXXXXXXX"
crossOrigin="anonymous"
/>
</Head>
<body>
<Main />
<NextScript />
</body>
</Html>
)
}
2. Creating an AdSense Component
Create a reusable component for your ads:
// components/AdSense.tsx
import { useEffect } from 'react'
type Props = {
adSlot: string
adFormat?: string
style?: React.CSSProperties
}
export const AdSense: React.FC<Props> = ({ adSlot, adFormat = 'auto', style }) => {
useEffect(() => {
try {
(window.adsbygoogle = window.adsbygoogle || []).push({})
} catch (err) {
console.error('AdSense error:', err)
}
}, [])
return (
<ins
className="adsbygoogle"
style={style || { display: 'block' }}
data-ad-client="ca-pub-XXXXXXXXXXXXXXXX"
data-ad-slot={adSlot}
data-ad-format={adFormat}
data-full-width-responsive="true"
/>
)
}
3. Implementation in Pages
Use the component in your pages:
import { AdSense } from '../components/AdSense'
export default function HomePage() {
return (
<div>
<h1>Welcome to my blog</h1>
<AdSense adSlot="1234567890" />
<article>
{/* Your content */}
</article>
</div>
)
}
Best Practices
- Make ads responsive
- Follow placement guidelines
- Optimize performance
- Implement lazy loading
- Test across devices
- Monitor performance
- Stay compliant with policies
Troubleshooting
Common issues to check:
- AdBlock interference
- Incorrect client ID
- JavaScript errors
- Pending approvals
- Mobile responsiveness
- Loading performance
Conclusion
Successful AdSense integration requires:
- Careful implementation
- Regular testing
- Policy compliance
- Performance monitoring
- User experience consideration
Follow these guidelines for effective monetization while maintaining site quality.