10 Advanced Tips to Reduce React Native Expo App Size
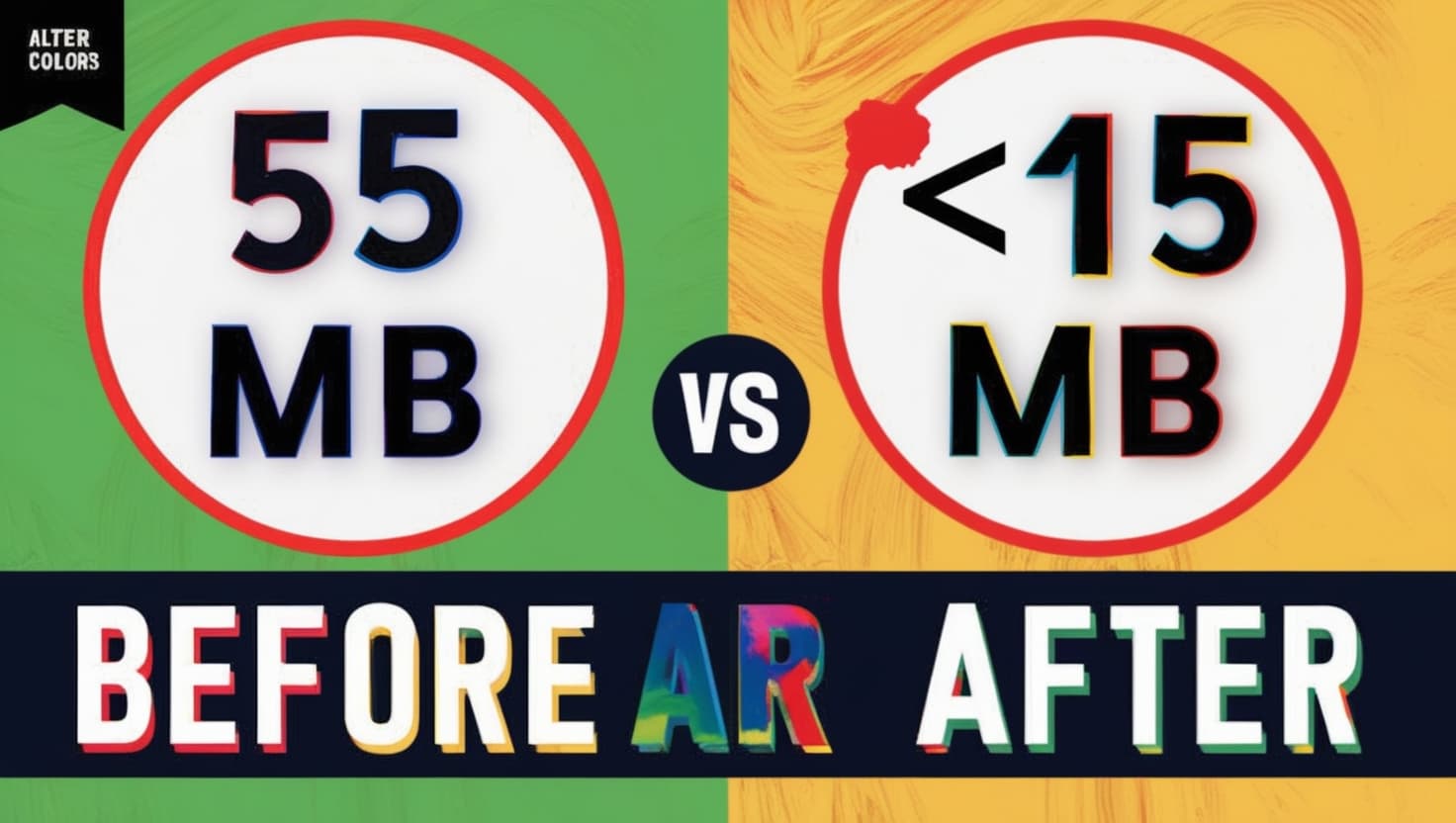
Table Of Content
- 10 Advanced Tips to Reduce React Native Expo App Size
- 1. Enable Proguard for Android
- 2. Implement Asset Management Strategy
- Optimize Images
- Load Assets Dynamically
- 3. Use Code Splitting
- 4. Optimize Dependencies
- Remove Unused Dependencies
- Use Lighter Alternatives
- 5. Configure Metro Bundler
- 6. Implement Font Loading Strategy
- 7. Use Production Builds
- 8. Implement Code Minification
- Configure Babel Optimization
- 9. Use Asset Preloading
- 10. Optimize Native Modules
- Remove Unused Expo Modules
- Best Practices for Implementation
- Results Measurement
- Conclusion
10 Advanced Tips to Reduce React Native Expo App Size
App size is crucial for user adoption and retention. Here are 10 advanced techniques to significantly reduce your React Native Expo app size.
1. Enable Proguard for Android
Proguard helps optimize and shrink your Android bundle by removing unused code:
// app.json
{
"expo": {
"android": {
"proguard": true
}
}
}
2. Implement Asset Management Strategy
Optimize Images
Use appropriate image formats and compression:
// Configure sharp for image optimization
const sharp = require('sharp');
async function optimizeImage(inputPath, outputPath) {
await sharp(inputPath)
.resize(800) // Set appropriate size
.jpeg({ quality: 80 })
.toFile(outputPath);
}
Load Assets Dynamically
const loadImage = async (imageName) => {
return await Asset.loadAsync(require(`../assets/${imageName}`));
};
3. Use Code Splitting
Implement dynamic imports for better code organization:
const MyComponent = React.lazy(() => import('./MyComponent'));
function App() {
return (
<Suspense fallback={<LoadingSpinner />}>
<MyComponent />
</Suspense>
);
}
4. Optimize Dependencies
Remove Unused Dependencies
Regularly audit and remove unnecessary packages:
npm audit
npm prune
Use Lighter Alternatives
Replace heavy libraries with lighter ones:
- Use day.js instead of moment.js
- Consider react-native-reanimated-lite instead of react-native-reanimated
5. Configure Metro Bundler
Create a custom metro config:
// metro.config.js
module.exports = {
transformer: {
minifierConfig: {
keep_classnames: false,
keep_fnames: false,
mangle: true,
compress: {
drop_console: true,
drop_debugger: true,
},
},
},
};
6. Implement Font Loading Strategy
Only load necessary font weights:
import * as Font from 'expo-font';
const loadFonts = async () => {
await Font.loadAsync({
'custom-regular': require('./assets/fonts/Custom-Regular.ttf'),
// Load only required weights
});
};
7. Use Production Builds
Enable production mode and remove development features:
// app.json
{
"expo": {
"web": {
"bundler": "metro"
},
"extra": {
"enableHermes": true
}
}
}
8. Implement Code Minification
Configure Babel Optimization
// babel.config.js
module.exports = function(api) {
api.cache(true);
return {
presets: ['babel-preset-expo'],
plugins: [
['transform-remove-console', { exclude: ['error', 'warn'] }]
]
};
};
9. Use Asset Preloading
Implement strategic asset preloading:
import { Asset } from 'expo-asset';
import * as Font from 'expo-font';
const cacheResources = async () => {
const imageAssets = [
require('./assets/logo.png'),
require('./assets/icon.png'),
];
const fontAssets = {
'custom-font': require('./assets/fonts/Custom.ttf'),
};
const imageLoadingPromises = imageAssets.map((image) =>
Asset.fromModule(image).downloadAsync()
);
const fontLoadingPromises = Font.loadAsync(fontAssets);
await Promise.all([...imageLoadingPromises, fontLoadingPromises]);
};
10. Optimize Native Modules
Remove Unused Expo Modules
// app.json
{
"expo": {
"plugins": [
[
"expo-build-properties",
{
"android": {
"excludeFromAndroidJar": [
"androidx.camera.core",
"androidx.camera.camera2"
]
}
}
]
]
}
}
Best Practices for Implementation
-
Regular Monitoring
- Use tools like
expo-doctor
to check app health - Monitor bundle size changes with each update
- Use tools like
-
Performance Testing
import { Performance } from 'expo-performance';
const measureStartup = async () => {
const metrics = await Performance.getMetrics();
console.log('App startup time:', metrics.startupTime);
};
- Continuous Optimization
- Regularly review and update optimization strategies
- Keep dependencies updated
- Monitor app performance metrics
Results Measurement
Track your optimization progress:
import * as Application from 'expo-application';
const logAppSize = async () => {
const size = await Application.getInstallationTimeAsync();
console.log('App installation size:', size);
};
Conclusion
Implementing these optimization techniques can significantly reduce your Expo app size. Remember to:
- Regularly monitor app size
- Test performance impact
- Balance size reduction with functionality
- Keep documentation updated
- Review optimization strategies periodically
For more information, check:
These optimizations can lead to:
- Faster download times
- Improved user adoption
- Better app store rankings
- Reduced storage requirements
- Enhanced overall performance
Remember to measure the impact of each optimization and maintain a balance between app size and functionality.