Best Practices for Typescript with nextjs 2024
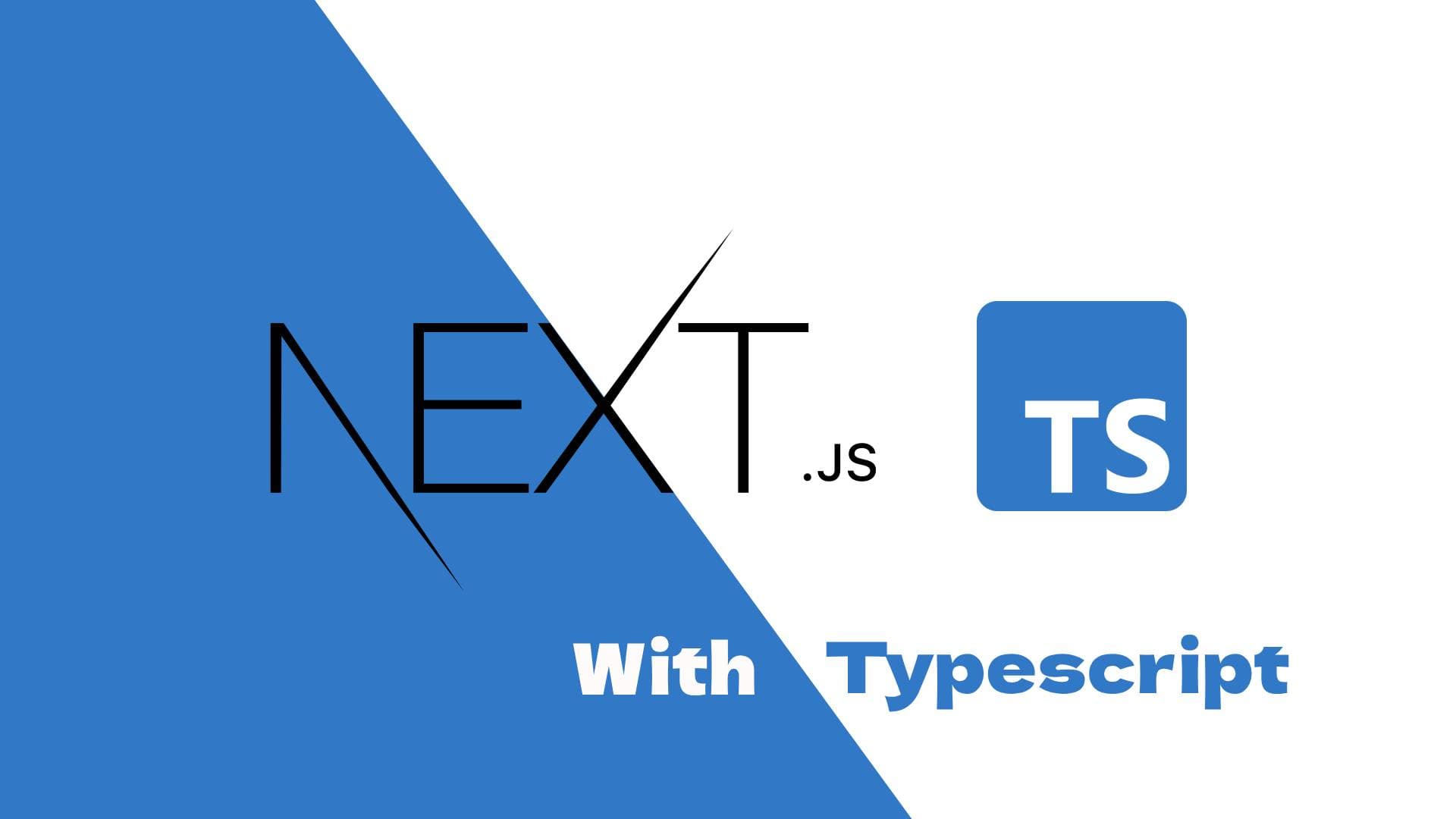
Essential TypeScript Features for Next.js Development
TypeScript has become an integral part of modern Next.js development, providing type safety and enhanced developer experience. This guide covers essential TypeScript features you need to know when building Next.js applications.
Key TypeScript Features for Next.js
1. Type Safety in Props
TypeScript allows you to define strict types for your component props, ensuring type safety across your application. Here's how to properly type your React components:
type Props = {
title: string;
description?: string;
children: React.ReactNode;
};
const Component = ({ title, description, children }: Props) => {
return (
<div>
<h1>{title}</h1>
{description && <p>{description}</p>}
{children}
</div>
);
};
2. API Route Types
Next.js provides built-in types for API routes. Here's how to type your API handlers:
import type { NextApiRequest, NextApiResponse } from 'next';
type Data = {
message: string;
};
export default function handler(
req: NextApiRequest,
res: NextApiResponse<Data>
) {
res.status(200).json({ message: 'Hello from API' });
}
3. GetStaticProps and GetServerSideProps
For data fetching methods, Next.js provides specific types:
import { GetStaticProps, GetServerSideProps } from 'next';
export const getStaticProps: GetStaticProps = async (context) => {
return {
props: {
data: 'your data'
}
};
};
Best Practices
- Use Strict Mode
Enable strict mode in your tsconfig.json:
{
"compilerOptions": {
"strict": true
}
}
- Type Your API Responses Create interfaces for API responses:
interface User {
id: number;
name: string;
email: string;
}
async function fetchUser(): Promise<User> {
const response = await fetch('/api/user');
return response.json();
}
- Leverage Path Aliases
Configure path aliases in tsconfig.json:
{
"compilerOptions": {
"baseUrl": ".",
"paths": {
"@components/*": ["components/*"],
"@lib/*": ["lib/*"]
}
}
}
Advanced Patterns
Generic Components:
type ListProps<T> = {
items: T[];
renderItem: (item: T) => React.ReactNode;
};
function List<T>({ items, renderItem }: ListProps<T>) {
return (
<ul>
{items.map((item, index) => (
<li key={index}>{renderItem(item)}</li>
))}
</ul>
);
}
Type Guards:
type Post = {
id: number;
title: string;
};
function isPost(obj: any): obj is Post {
return 'id' in obj && 'title' in obj;
}
Conclusion
Mastering TypeScript in Next.js development is crucial for building robust applications. These features and patterns will help you write more maintainable and type-safe code.
Remember to:
- Always define proper types for your components and functions
- Use TypeScript's built-in utility types
- Leverage Next.js specific types
- Keep your tsconfig.json properly configured
By following these guidelines, you'll create more reliable and maintainable Next.js applications.